After several months working on various Rhino + Grasshopper plugins and developing new UI for the custom components in grasshopper , I just decided to package all controls into a C# library and make it available to all Grasshopper developers. The current version includes several interactive controls which can be easily added to the GH component by implementing an abstract class called
GHCustomComponent
The complete documentation will follow soon, however, here is a brief “how to use” for whoever wants to put his hands on. If you wish to have access to the shared library please get in touch !
In order to use the controls you first need to inherit from the abstract class GHCustomComponent and then create and add your controls when the RegisterInputParams()method is called.
See the sample code for Declaration , Creation and Registering a custom control in below.
When it comes to retrieving the value of the control simply check the CurrentValue property as it is shown in the next sample code.
All the interactions and graphics are taken care so it must be easy for the end user to integrate the controls and use them through his code.
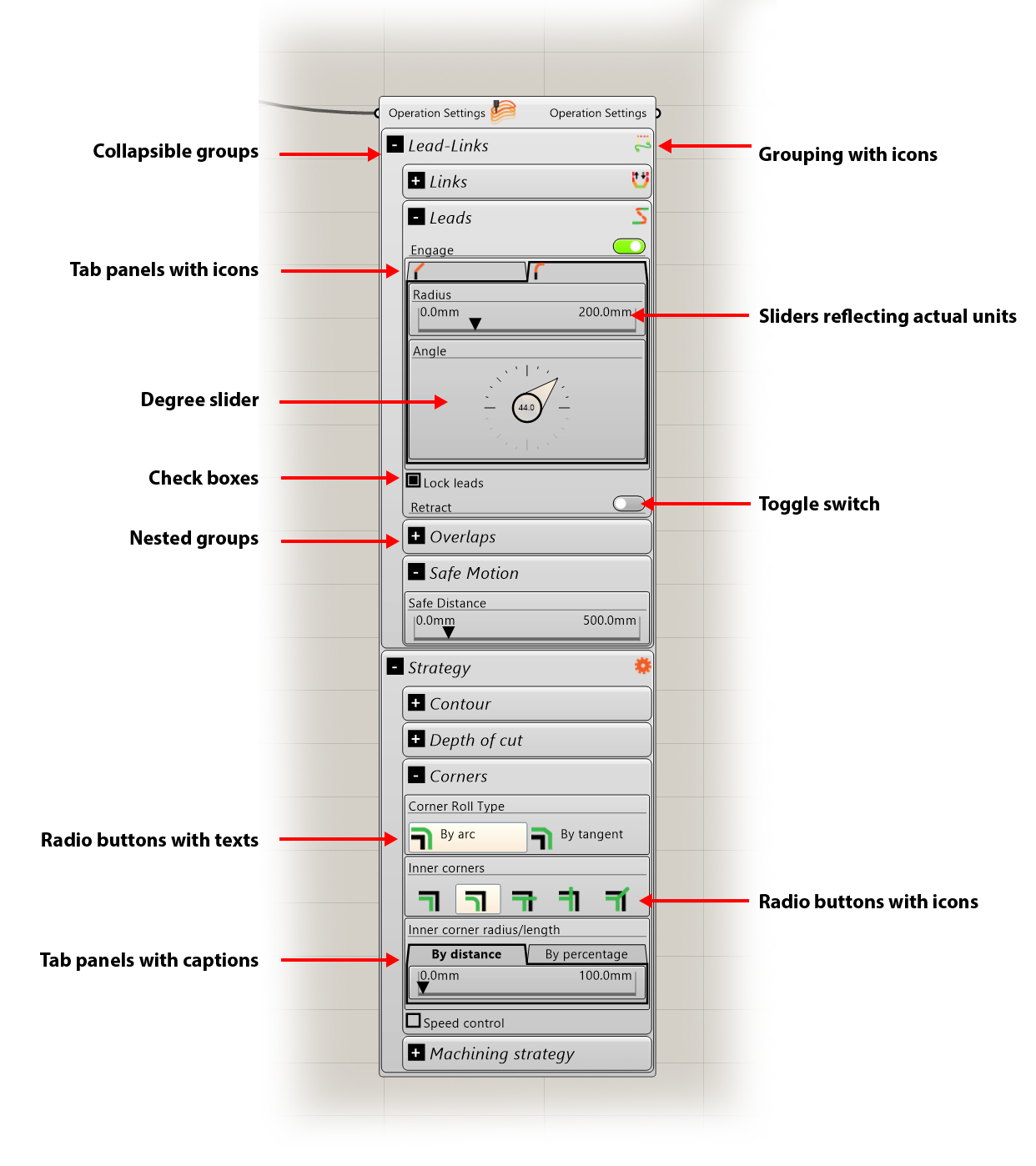
Declaration , Creation and Registering a custom control
using System; using System.Collections.Generic; using Grasshopper.Kernel; using Rhino.Geometry; using GHCustomControls; // In order to load the result of this wizard, you will also need to // add the output bin/ folder of this project to the list of loaded // folder in Grasshopper. // You can use the _GrasshopperDeveloperSettings Rhino command for that. namespace GHCustomControlDemo { public class GHCustomControlDemoComponent : GHCustomComponent { /// <summary> /// Declare the check box /// </summary> CheckBox checkBox; /// <summary> /// Each implementation of GH_Component must provide a public /// constructor without any arguments. /// Category represents the Tab in which the component will appear, /// Subcategory the panel. If you use non-existing tab or panel names, /// new tabs/panels will automatically be created. /// </summary> public GHCustomControlDemoComponent() : base("GHCustomControlDemo", "Nickname", "Description", "Category", "Subcategory") { } /// <summary> /// Registers all the input parameters for this component. /// </summary> protected override void RegisterInputParams(GH_Component.GH_InputParamManager pManager) { // create the checkbox checkBox = new CheckBox("MyCheckBox","This is a test",false); //register your input parameters as usual //.... //.... // register the check box. AddCustomControl(checkBox); }
Retrieving the value of the control.
protected override void SolveInstance(IGH_DataAccess DA) { //read the check box status bool checkBoxStatus = (bool)checkBox.CurrentValue; }